Using WebView with Flutter
Flutter comes with many packages that grant the possibility to run web pages in mobile apps. After research, the leader has been found: webview_flutter.
Why webview_flutter
First of all, it is currently maintained by the official Google team. When the development started, there were no official recommendations by the Flutter team on which package should be used.
The second reason why we started using this package — it gives us the possibility to share data between the web page and the flutter application in two directions. This feature was crucial when we investigated this case.
On iOS the WebView widget is backed by a WKWebView; On Android, the WebView widget is backed by a WebView.
How to set up webview_flutter
The setup is pretty easy. Install the library using the description on the official page.
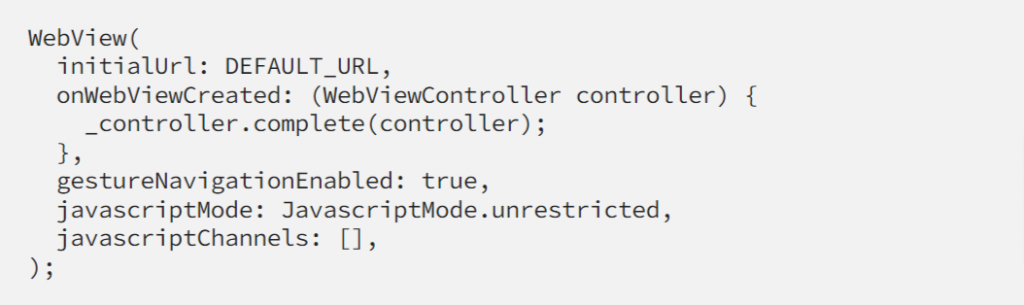
How do we get data from a web page
JavascriptChannel gives us possibility to get data from web page. We set it up in the javascriptChannels
list:

Firstly we choose the name for the channel in name
parameter. This name will used to access channel from inside the web page. When we call the method channel from the page, onMessageReceived will be fired transporting the message
.
Now let’s see how to send messages from the page. Firstly, webview_flutter
mutates window
object. If a web page has been loaded using this package it will have a property that we have defined in JavascriptChannel
. In our case we can access the channel by calling:

We can use postMessage
method to pass data to WebView and trigger onMessageReceived
callback.
If you should use TypeScript in your project, you will need to override Window
interface using following syntax:
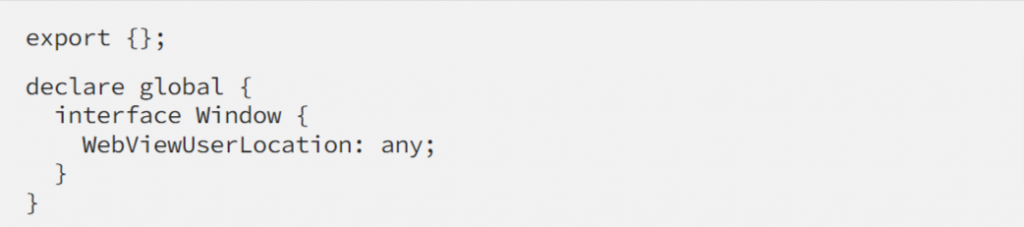
How to pass data to a web page
In this particular case, we can not just use a JavascriptChannel
, we should somehow inject some JavaScript code that will fire messages inside the web page. In this case, the web page will have a subscriber that will process the data received from the app.
The packagewebview_flutter
comes with a solution. We can use WebViewController
class that has runJavascript(String script)
method:

Once script is executed and message is fired, a callback from inside the page is triggered:

Summary
In this article we have successfully transported data from a web page into Flutter application using WebView and vice versa.
Web page:
Send data: window.WebViewUserLocation.postMessage('')
;
Receive data: window.addEventListener('message', onMessageReceived);
Flutter app:
Send data:

Receive data: use JavascriptChannel